알아두면 유용한 7가지 파이썬 표준 라이브러리 함수
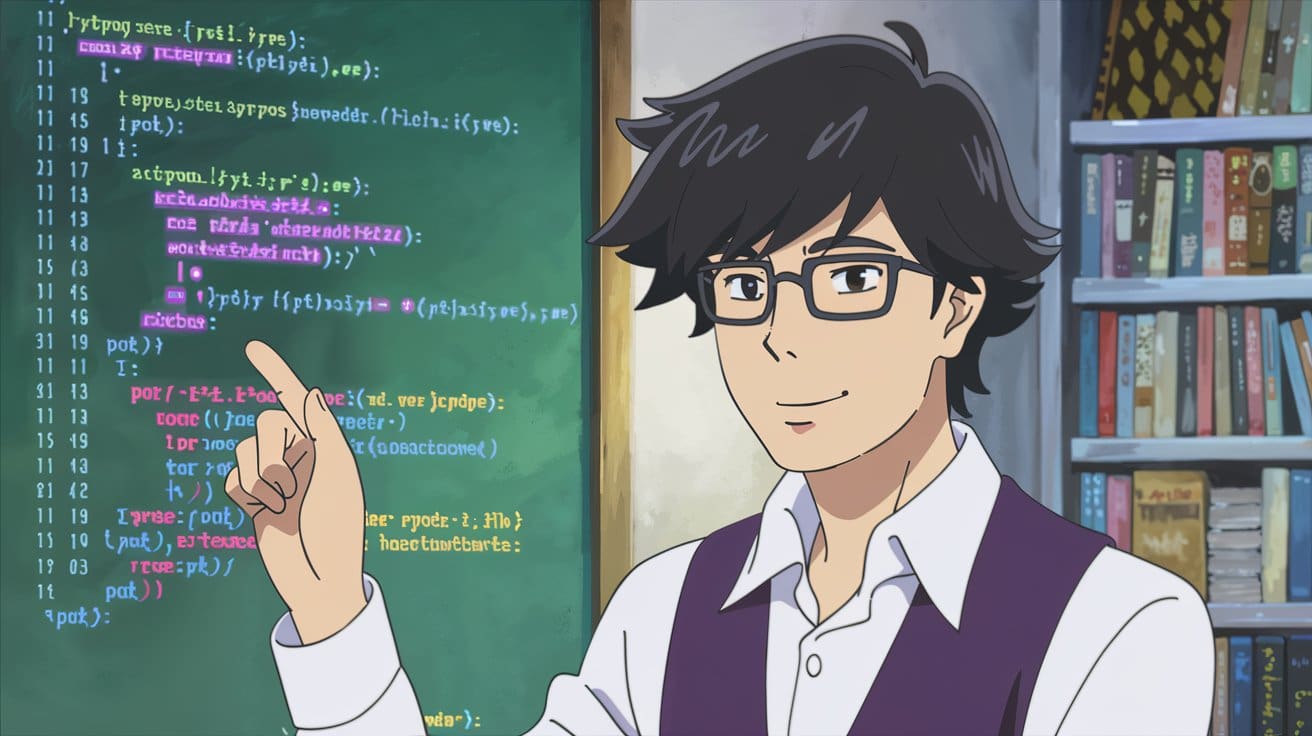
파이썬을 사용해 본 사람이라면 개발을 효율적이고 간단하게 만들어주는 방대한 표준 라이브러리에 익숙할 것입니다. json, datetime, re와 같은 인기 모듈들이 많은 주목을 받는 반면, 덜 알려진 함수들도 있습니다.
이 글에서는 그런 함수들, 즉 "쓸모없어 보이는" 함수들에 대해 알아보겠습니다. 하지만 사실 이 함수들은 전혀 쓸모없지 않습니다! 오히려 특정 상황에서 매우 유용하게 사용될 수 있습니다.
1. textwrap.dedent() - 복잡한 여러 줄 문자열 정리하기
여러 줄 문자열을 작성할 때 들여쓰기로 인해 불편함을 느낀 적이 있나요? 그렇다면 textwrap.dedent
가 도움이 될 것입니다!
다음 예제는 textwrap의 dedent
를 사용합니다. 이 함수는 소스 코드에서 들여쓰기된 여러 줄 문자열에서 불필요한 들여쓰기를 제거하고 깔끔한 텍스트를 만들어 줍니다.
import textwrap
def my_function():
# Without dedent, this would preserve all the leading spaces
description = textwrap.dedent("""
This is a multi-line string
that will have consistent indentation
regardless of how it's indented in the code.
Pretty neat, right?
""").strip()
return description
print(my_function())
출력:
This is a multi-line string
that will have consistent indentation
regardless of how it's indented in the code.
Pretty neat, right?
2. difflib.getclosematches() - 쉬운 퍼지 문자열 매칭
문자열 간 유사성을 찾거나 "이것을 찾으셨나요?" 기능을 구현하고 싶을 때, difflib 모듈의 getclosematches
함수가 유용합니다.
다음은 이 함수를 사용하는 예제입니다:
import difflib
words = ["python", "javascript", "typescript", "ruby", "golang"]
search = "pythn"
matches = difflib.getclosematches(search, words, n=3, cutoff=0.6)
print(f"Did you mean: {matches}")
출력:
Did you mean: ['python']
첫 번째 예제는 프로그래밍 언어 목록에서 "pythn"과 가장 가까운 단어를 찾습니다.
두 번째 예제는 "typescript"와 유사한 단어로 "typescript"와 "javascript"가 모두 포함됨을 보여줍니다.
search = "typescript"
matches = difflib.getclosematches(search, words)
print(f"Matches: {matches}")
출력:
['typescript', 'javascript']
이 기능은 명령줄 도구, 검색 기능 또는 오타나 유사한 단어를 고려해야 하는 상황에서 특히 유용합니다.
3. uuid.uuid4() - 고유 ID 생성하기
데이터베이스 설정이나 충돌 걱정 없이 고유 식별자가 필요하다면 uuid 모듈의 함수를 사용할 수 있습니다.
다음 코드는 고유 식별자로 사용할 수 있는 무작위 UUID 객체를 생성합니다:
import uuid
Generate a random UUID
random_id = uuid.uuid4()
print(f"Unique ID: {random_id}")
출력:
Unique ID: fc4c6638-9707-437b-83a1-76206b5f7191
다음 예제는 파일 이름에 UUID를 통합하여 고유성을 보장하는 방법을 보여줍니다:
Use as string for filenames, database keys, etc.
filename = f"document-{uuid.uuid4()}.pdf"
print(filename)
출력:
document-b5ccbe7a-fad9-4611-8163-be1015c634b9.pdf
이러한 UUID(Universally Unique Identifier)는 서로 다른 기계와 시간에도 고유성이 보장되므로 파일, 데이터베이스 항목 또는 고유성이 필요한 곳에 ID를 생성하는 데 완벽합니다.
4. shutil.getterminalsize() - 반응형 CLI 애플리케이션
명령줄 애플리케이션이 사용자의 터미널 크기에 맞게 조정되길 원하나요? getterminalsize
함수가 쉽게 해결해 줍니다.
사용 예:
import shutil
columns, rows = shutil.getterminalsize()
print(f"Your terminal is {columns} columns wide and {rows} rows tall")
Create a horizontal divider that fits perfectly
print("-" * columns)
이 코드는 터미널의 현재 크기를 열과 행으로 가져오고, 터미널 너비에 정확히 맞는 수평 구분선을 만듭니다.
Your terminal is 80 columns wide and 24 rows tall
--------------------------------------------------------------------------------
5. itertools.groupby() - 딕셔너리 없이 데이터 그룹화하기
어떤 키로 데이터를 그룹화해야 할 때, itertools.groupby()
를 사용하면 효율적으로 처리할 수 있습니다.
다음 예제에서는 직원 목록을 부서별로 먼저 정렬한 후(groupby가 제대로 작동하려면 필요) 부서별로 그룹화합니다. 그런 다음 부서별로 그룹화된 각 직원을 출력합니다.
from itertools import groupby
from operator import itemgetter
Sample data: (name, department)
employees = [
("Alice", "Engineering"),
("Bob", "Marketing"),
("Charlie", "Engineering"),
("Diana", "HR"),
("Evan", "Marketing"),
]
Sort by department first (groupby works on consecutive items)
employees.sort(key=itemgetter(1))
Group by department
for department, group in groupby(employees, key=itemgetter(1)):
print(f"\n{department} Department:")
for name, _ in group:
print(f" - {name}")
출력:
Engineering Department:
- Alice
- Charlie
HR Department:
- Diana
Marketing Department:
- Bob
- Evan
6. collections.ChainMap - 부담 없이 딕셔너리 병합하기
여러 딕셔너리를 검색해야 하나요? ChainMap
을 사용하면 실제로 병합하지 않고도 순서대로 검색할 수 있습니다.
참고: 이것은 함수가 아니라 Python 표준 라이브러리의 collections 모듈에 있는 유용한 클래스입니다.
실용적인 예제:
from collections import ChainMap
defaults = {"theme": "dark", "language": "en", "timeout": 30}
user_settings = {"theme": "light"}
session_settings = {"timeout": 60}
Create a combined view of all settings
settings = ChainMap(sessionsettings, usersettings, defaults)
print(settings["theme"])
print(settings["language"])
print(settings["timeout"])
이는 딕셔너리를 병합하지 않고 순서대로 값을 조회하는 여러 딕셔너리의 뷰를 만들며, 서로 다른 소스의 설정 우선순위를 지정하는 방법을 보여줍니다.
출력:
light
en
60
7. os.path.commonpath() - 공유 디렉토리 경로 찾기
여러 파일 경로 간의 공통 디렉토리를 찾아야 했던 적이 있나요? os 모듈의 path.commonpath
를 사용하면 정확히 그 작업을 수행할 수 있습니다.
다음 코드는 파일 경로 목록에서 가장 긴 공통 디렉토리 경로를 식별하며, 파일 그룹의 기본 디렉토리를 찾는 데 유용합니다:
import os.path
paths = [
"/home/user/documents/work/report.pdf",
"/home/user/documents/personal/taxes.xlsx",
"/home/user/documents/work/presentation.pptx"
]
common = os.path.commonpath(paths)
print(f"Common directory: {common}")
출력:
Common directory: /home/user/documents
이 함수는 파일 세트의 공통 루트 디렉토리를 찾거나 상대 경로를 구축하는 것과 같은 작업에 매우 유용합니다.
마무리
살펴본 바와 같이, Python의 표준 라이브러리에는 일반적으로 사용되지는 않지만 특정 문제에 대한 우아한 해결책을 제공하는 여러 특수 함수가 있습니다.
다음에 일반적인 문제를 해결하기 위해 복잡한 함수를 작성할 때는 표준 라이브러리에 이미 해결책이 있는지 확인해 보세요!