Lesser-Known Python Functions That Are Super Useful
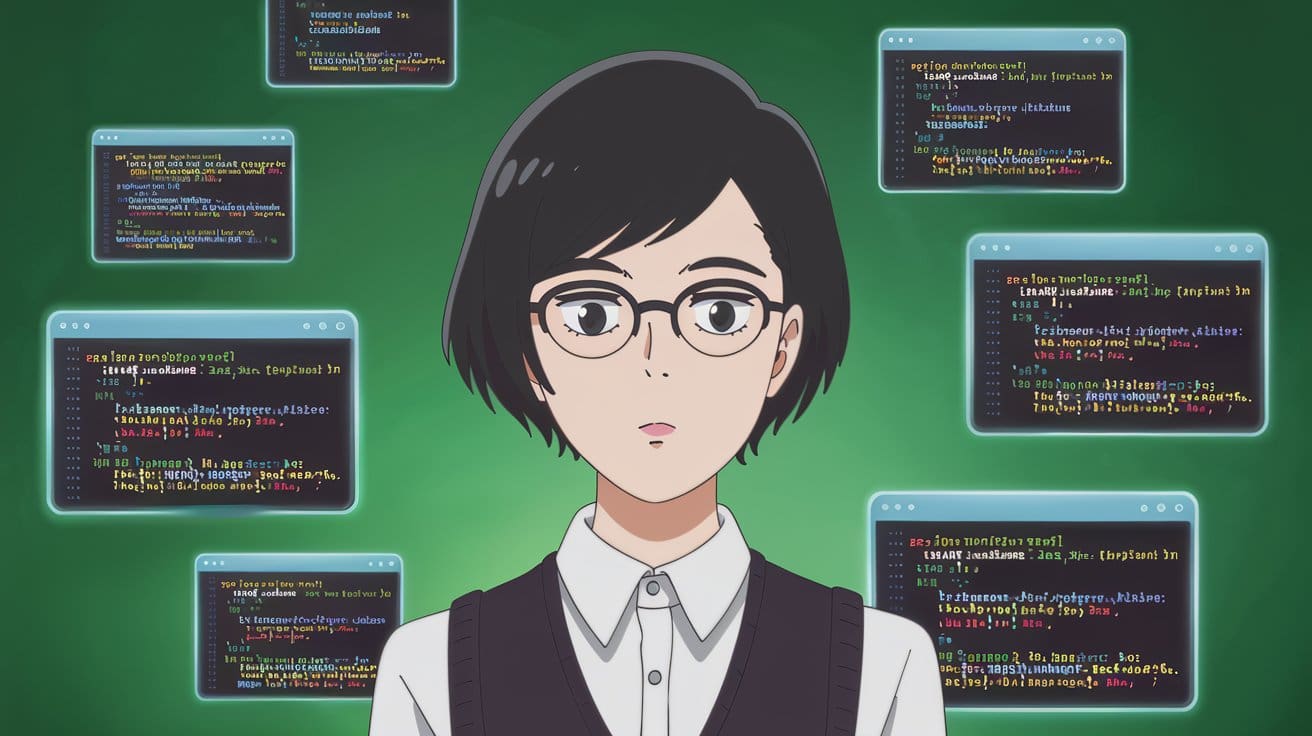
As developers, we often get comfortable with our go-to built-in Python functions and even jump right into writing our own functions for non-trivial tasks. Python's standard library, however, is packed with some super helpful functions that often fly under the radar.
In this article, we'll look at a collection of underutilized Python functions that deserve way more spotlight. Let's get started right away!
1. bisect
Python's built-in bisect module
is super useful when you need to work with sorted sequences. It provides functions for maintaining sorted lists efficiently and finding insertion points quickly.
In this example, we code a practical grade tracking system that shows three key uses of bisect:
- finding insertion points,
- maintaining sorted lists, and
from bisect import bisectleft, bisectright, insort
Let's create a grade tracking system
grades = [60, 70, 75, 85, 90, 95]
Find where to insert a new grade while keeping the list sorted
new_grade = 82
position = bisectleft(grades, newgrade)
print(f"Insert 82 at position: {position}")
Insert while maintaining sort order
insort(grades, new_grade)
print(f"Grades after insertion: {grades}")
Find grade ranges
def gradetoletter(score):
breakpoints = [60, 70, 80, 90] # F, D, C, B, A
grades = 'FDCBA'
position = bisect_right(breakpoints, score)
return grades[position]
print(f"Score 82 gets grade: {gradetoletter(82)}")
print(f"Score 75 gets grade: {gradetoletter(75)}")
Output:
Insert 82 at position: 3
Grades after insertion: [60, 70, 75, 82, 85, 90, 95]
Score 82 gets grade: B
Score 75 gets grade: C
This is particularly efficient for use cases where traditional methods would require multiple comparisons.
2. itertools.pairwise
The pairwise
function from the itertools module is useful when you need to process consecutive pairs in a sequence. This is particularly useful for analyzing trends or calculating differences.
Let's take a practical example of temperature analysis. With the pairwise function, we can calculate changes between readings:
from itertools import pairwise
Let's analyze temperature changes
temperatures = [20, 23, 24, 25, 23, 22, 20]
Calculate temperature changes between consecutive readings
changes = []
for prev, curr in pairwise(temperatures):
change = curr - prev
changes.append(change)
print("Temperature changes:", changes)
Output:
Temperature changes: [3, 1, 1, -2, -1, -2]
You can also compute moving averages:
Calculate moving averages
moving_averages = []
for t1, t2 in pairwise(temperatures):
avg = (t1 + t2) / 2
moving_averages.append(avg)
print("Moving averages:", moving_averages)
Output:
Moving averages: [21.5, 23.5, 24.5, 24.0, 22.5, 21.0]
And tasks like finding the largest temperature jump:
Finding the largest temperature jump
max_jump = max(abs(b - a) for a, b in pairwise(temperatures))
print(f"Largest temperature change: {max_jump} degrees")
Output:
Largest temperature change: 3 degrees
This function eliminates the need for complex indexing or manual iteration that often leads to off-by-one errors.
3. statistics.fmean
The fmean
function from the built-in statistics module is both faster and more precise than the usual mean()
. It's particularly useful when working with floating-point numbers or large datasets.
from statistics import mean, fmean
import time
Let's compare fmean with regular mean
Imagine we're analyzing daily temperature readings
temperatures = [
21.5, 22.1, 23.4, 22.8, 21.8,
23.2, 22.7, 23.1, 22.6, 21.9
] * 100000 # Create a large dataset
Let's compare speed and precision
starttime = time.perfcounter()
regular_mean = mean(temperatures)
regulartime = time.perfcounter() - start_time
starttime = time.perfcounter()
fast_mean = fmean(temperatures)
fasttime = time.perfcounter() - start_time
print(f"Regular mean: {regularmean:.10f} (took {regulartime:.4f} seconds)")
print(f"fmean: {fastmean:.10f} (took {fasttime:.4f} seconds)")
Output:
Regular mean: 22.5100000000 (took 0.4748 seconds)
fmean: 22.5100000000 (took 0.0164 seconds)
You'll notice fmean is significantly faster and maintains precision. The difference is especially beneficial with larger datasets.
4. itertools.takewhile
Itertools module takewhile
is useful for processing sequences until a condition is no longer met. It's a cleaner version of breaking a loop when a condition fails.
Let's take this example of processing log entries until an error occurs.
from itertools import takewhile
Processing log entries until an error
log_entries = [
"INFO: System started",
"INFO: Loading data",
"INFO: Processing users",
"ERROR: Database connection failed",
"INFO: Retrying connection",
]
Get all logs until first error
normal_operation = list(takewhile(
lambda x: not x.startswith("ERROR"),
log_entries
))
print("Logs before first error:")
for entry in normal_operation:
print(entry)
Output:
Logs before first error:
INFO: System started
INFO: Loading data
INFO: Processing users
5. operator.attrgetter
It can often be difficult to efficiently access nested attributes of objects, especially when dealing with large datasets or complex object structures. The attrgetter
function solves this by providing a memory-efficient way to retrieve nested attributes and creates reusable accessor functions.
Let's code an example.
from operator import attrgetter
from datetime import datetime
Let's create a simple class to demonstrate
class Article:
def init(self, title, author, views, date):
self.title = title
self.author = author
self.stats = type('Stats', (), {'views': views}) # Nested attribute
self.date = date
def repr(self):
return f"{self.title} by {self.author}"
Create some sample articles
articles = [
Article("Python Tips", "Alice", 1500, datetime(2025, 1, 15)),
Article("Data Science", "Bob", 2500, datetime(2025, 1, 20)),
Article("Web Dev", "Alice", 1800, datetime(2025, 1, 10))
]
We can now sort the articles as needed.
Sort articles by multiple criteria
getauthorviews = attrgetter('author', 'stats.views')
Sort by author and then by views
sortedarticles = sorted(articles, key=getauthor_views)
for article in sorted_articles:
print(f"{article.author}: {article.title} ({article.stats.views} views)")
You can also use it to extract specific attributes
dates = list(map(attrgetter('date'), articles))
print("\nArticle dates:", dates)
Output:
Alice: Python Tips (1500 views)
Alice: Web Dev (1800 views)
Bob: Data Science (2500 views)
Article dates: [datetime.datetime(2025, 1, 15, 0, 0), datetime.datetime(2025, 1, 20, 0, 0), datetime.datetime(2025, 1, 10, 0, 0)]
In the example above, we demonstrate this by working with a blog article system where we need to sort and access various nested properties.
6. itertools.chain.from_iterable
When working with nested data structures (lists within lists, or any nested iterables), flattening them efficiently can be challenging.
While list comprehensions are common, they create intermediate lists that consume memory. chain.from_iterable
solves this by providing a memory-efficient way to flatten nested structures by creating an iterator.
from itertools import chain
Let's say we're processing data from multiple sources
sales_data = [
[('Jan', 100), ('Feb', 150)],
[('Mar', 200), ('Apr', 180)],
[('May', 210), ('Jun', 190)]
]
Flatten the data efficiently
flatsales = list(chain.fromiterable(sales_data))
print("Flattened sales data:", flat_sales)
List comprehension approach (creates intermediate list):
flatlist = [item for sublist in salesdata for item in sublist]
chain.from_iterable approach (generates items one at a time):
flatiterator = chain.fromiterable(sales_data)
Output:
Flattened sales data: [('Jan', 100), ('Feb', 150), ('Mar', 200), ('Apr', 180), ('May', 210), ('Jun', 190)]
7. itertools.product
You can use the itertools.product
function for generating all possible combinations of input iterables.
Let's see how the product function can help with generating combinations say you're building a customization system for a product.
from itertools import product
Available options for a custom laptop
processors = ['i5', 'i7', 'i9']
ram = ['8GB', '16GB', '32GB']
storage = ['256GB', '512GB', '1TB']
Generate all possible combinations
configurations = list(product(processors, ram, storage))
print("Possible laptop configurations:")
for config in configurations:
print(f"Processor: {config[0]}, RAM: {config[1]}, Storage: {config[2]}")
Output:
Possible laptop configurations:
Processor: i5, RAM: 8GB, Storage: 256GB
Processor: i5, RAM: 8GB, Storage: 512GB
Processor: i5, RAM: 8GB, Storage: 1TB
Processor: i5, RAM: 16GB, Storage: 256GB
Processor: i5, RAM: 16GB, Storage: 512GB
Processor: i5, RAM: 16GB, Storage: 1TB
Processor: i5, RAM: 32GB, Storage: 256GB
Processor: i5, RAM: 32GB, Storage: 512GB
Processor: i5, RAM: 32GB, Storage: 1TB
.
.
.
Processor: i9, RAM: 32GB, Storage: 256GB
Processor: i9, RAM: 32GB, Storage: 512GB
Processor: i9, RAM: 32GB, Storage: 1TB
This function eliminates the need for nested loops and makes the code more readable and maintainable.
요약
여기 살펴본 모든 함수들을 빠르게 정리해보겠습니다:
- bisect – 정렬된 시퀀스를 효율적으로 유지하기 위한 함수
- itertools.pairwise – 연속된 데이터 쌍을 처리하기 위한 함수
- statistics.fmean – 더 정확한 평균 계산 함수
- itertools.takewhile – 조건이 충족될 때까지 시퀀스를 처리하는 함수
- operator.attrgetter – 중첩된 속성에 더 깔끔하게 접근하기 위한 함수
- itertools.chain.from_iterable – 중첩된 이터러블을 깔끔하게 평탄화하는 함수